In this article, we will discuss how to write a multi-line macro. We can write multi-line macro same like function, but each statement ends with “ ”. Let us see with example. Below is simple macro, which accepts input number from user, and prints whether entered number is even or odd. Using the Code from Excel Macro Examples. Here are the steps you need to follow to use the code from any of the examples: Open the Workbook in which you want to use the macro. Hold the ALT key and press F11. This opens the VB Editor. Right-click on any of the objects in the project explorer. Go to Insert.
- C++ Basics
- C++ Object Oriented
C Macro Example Definition
- C++ Advanced
- C++ Useful Resources
- Selected Reading
The preprocessors are the directives, which give instructions to the compiler to preprocess the information before actual compilation starts.
All preprocessor directives begin with #, and only white-space characters may appear before a preprocessor directive on a line. Preprocessor directives are not C++ statements, so they do not end in a semicolon (;).
You already have seen a #include directive in all the examples. This macro is used to include a header file into the source file.
There are number of preprocessor directives supported by C++ like #include, #define, #if, #else, #line, etc. Let us see important directives −
The #define Preprocessor
The #define preprocessor directive creates symbolic constants. The symbolic constant is called a macro and the general form of the directive is −
When this line appears in a file, all subsequent occurrences of macro in that file will be replaced by replacement-text before the program is compiled. For example −
Now, let us do the preprocessing of this code to see the result assuming we have the source code file. So let us compile it with -E option and redirect the result to test.p. Now, if you check test.p, it will have lots of information and at the bottom, you will find the value replaced as follows −
Function-Like Macros
You can use #define to define a macro which will take argument as follows −
If we compile and run above code, this would produce the following result −
C Define Macro Function Example
Conditional Compilation
There are several directives, which can be used to compile selective portions of your program's source code. This process is called conditional compilation.
The conditional preprocessor construct is much like the ‘if’ selection structure. Consider the following preprocessor code −
You can compile a program for debugging purpose. You can also turn on or off the debugging using a single macro as follows −
This causes the cerr statement to be compiled in the program if the symbolic constant DEBUG has been defined before directive #ifdef DEBUG. You can use #if 0 statment to comment out a portion of the program as follows −
Let us try the following example −
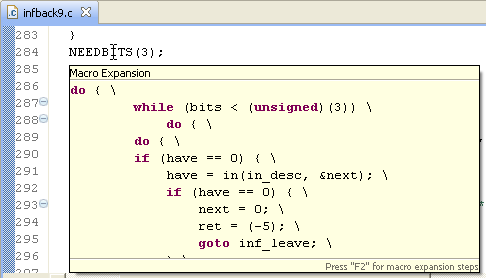
If we compile and run above code, this would produce the following result −
The # and ## Operators
The # and ## preprocessor operators are available in C++ and ANSI/ISO C. The # operator causes a replacement-text token to be converted to a string surrounded by quotes.
Consider the following macro definition −
If we compile and run above code, this would produce the following result −
Let us see how it worked. It is simple to understand that the C++ preprocessor turns the line −
Above line will be turned into the following line −
The ## operator is used to concatenate two tokens. Here is an example −
When CONCAT appears in the program, its arguments are concatenated and used to replace the macro. For example, CONCAT(HELLO, C++) is replaced by 'HELLO C++' in the program as follows.
If we compile and run above code, this would produce the following result −
Let us see how it worked. It is simple to understand that the C++ preprocessor transforms −
Above line will be transformed into the following line −
Predefined C++ Macros
C++ provides a number of predefined macros mentioned below −
Sr.No | Macro & Description |
---|---|
1 | __LINE__ This contains the current line number of the program when it is being compiled. |
2 | __FILE__ This contains the current file name of the program when it is being compiled. |
3 | __DATE__ This contains a string of the form month/day/year that is the date of the translation of the source file into object code. |
4 | __TIME__ This contains a string of the form hour:minute:second that is the time at which the program was compiled. |
C Preprocessor Macros
Let us see an example for all the above macros −

C Assert Macro Example
If we compile and run above code, this would produce the following result −